2.
#include <lua5.2/lua.h>
3.
#include <lua5.2/lualib.h>
4.
#include <lua5.2/lauxlib.h>
Great, Lua is now included in your project. You can call any of the functions provided. Lets go ahead and set up the Lua state.
8.
// Create a new Lua state
9.
lua_State *L = luaL_newstate();
10.
11.
// Initialize all default Lua libraries
12.
luaL_openlibs(L);
Firstly, we create the new Lua state by calling luaL_newstate()
Next, we initialize all of the default Lua libraries by calling luaL_openlibs(L)
Now that the Lua state is open, you may call functions like lua_getglobal
and lua_pushstring
14.
// Load the script
15.
if (luaL_loadfile(L, "player.lua") != LUA_OK) {
16.
printf("lua: %s\n", lua_tostring(L, -1));
17.
}
18.
19.
// Execute the script
20.
if (lua_pcall(L, 0, 0, 0)) {
21.
printf("lua %s\n", lua_tostring(L, -1));
22.
}
Congradulations, the script player.lua
has now run. If there were any errors, they would've been printed to stdout. Now that the script has run, we can call the following to cleanup the Lua state.
24.
// Close the Lua state
25.
lua_close(L);
gcc embeddinglua.c -llua
If you wish to download the full source for this tutorial, it can be found here.
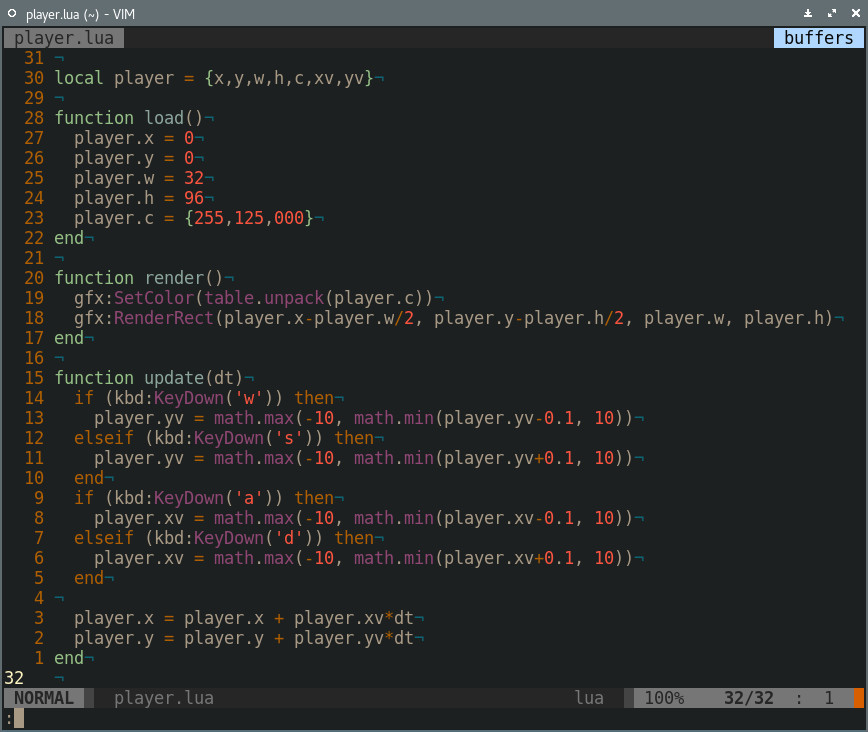